My air quality sensors are coming along nicely and sending data to multiple data stores works very well over WiFi, so the next challenge to solve is sending the data over a mobile network so they can be deployed into remote locations.
Tara from the WildLabs Slack group mentioned that Safecast were experimenting with a new LTE board, a Blues Notecard. Blues offering is a low-power, easy to use/integrate solution for sending small packets of data over LTE. Perfect.
I wasn’t expecting to hear back so quickly from the Blues team, but within a week I had a dev kit ready to test.
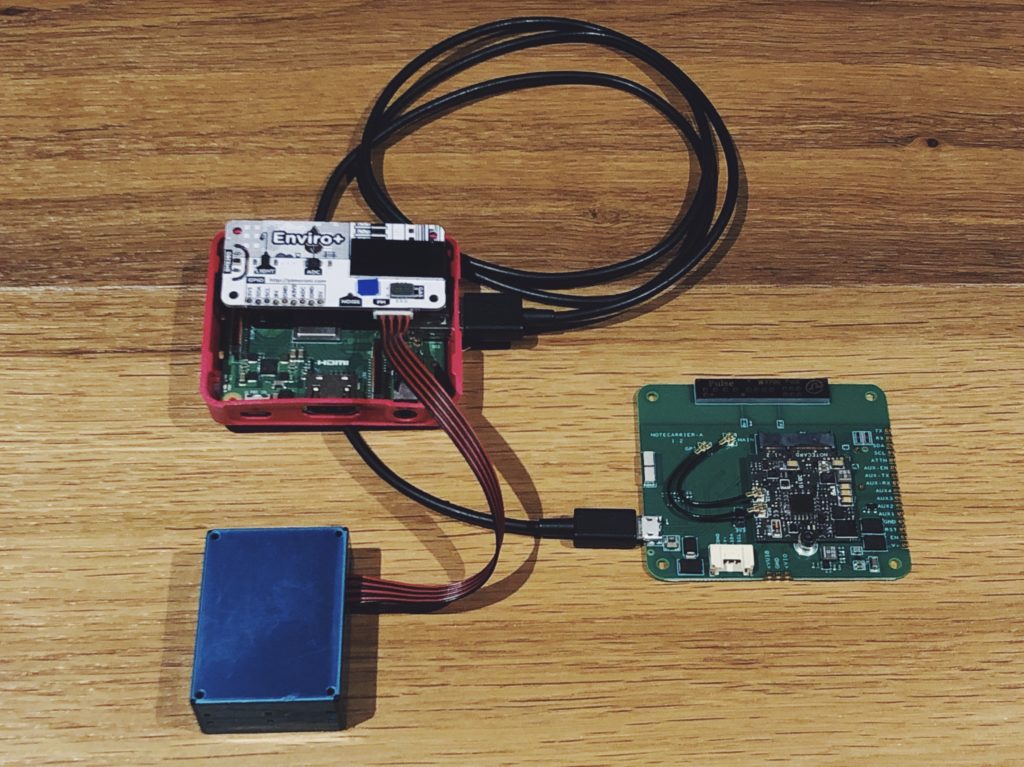
Getting started
The Blues Notecard comes with the ability to connect with it over USB, I2C or UART. Given that my Enviro+ sensors are using all of the onboard I2C and UART, my only option was to connect via USB – which was actually handy as I could use that for powering the Notecard too.
Setup was as easy as plugging in the micro-USB to my Raspberry Pi A+ USB port, and LEDs lit up!
The Notecard has a CLI (command line interface) tool to get started for Linux, macOS or Windows. I decided to have a play with this first to confirm that the hardware was working.
$ ./notecard -info
Blues Wireless Notecard
ProductUID: com.XXX.demo
Serial Number:
DeviceUID: dev:example
Notehub Host: a.notefile.net
Version: notecard-1.2.3.9536
Modem: XXXXXXXX
ICCID: XXXXXXXX
IMSI: XXXXXXXX
IMEI: XXXXXXXX
Provisioned:
Used Over-the-Air: 0 bytes
Sync Mode: periodic
Sync Upload Period: 0 mins
Download Period: 0 hours
Notehub Status:
Last Synced:
Voltage: 4.76V
Temperature: 13.69C
GPS Mode: off (GPS is disabled)
Location:
Currently: 2020-05-02T17:04:18Z (2020-05-02 5:04:18 PM ACST)
Booted:
Notefiles:
Notefile Storage Used: 0%
Env:
It’s so nice to receive hardware that Just Works™️ out of the box. I literally just plugged it in and ran the CLI. No soldering, no uploading firmware, no port configuring, no sim card purchase. Amazing.
Next step was to send my first piece of data. I was a little sceptical about how that’d go given that I was in Port Willunga with pretty average cell tower reception even on my phone, but worth a try. The beauty of interfacing with the Notecard is that you simply send it JSON with a body filled with your sensor data.
$ ./notecard -play
You may now enter Notecard JSON requests interactively.
Show sync activity by using "w" to toggle Watch Mode on/off.
> {"req":"note.add","file":"mydata.qo","body":{"sensor1":6, "units":"PM2.5 ug/m3"}}
{"total":1}
> {"req":"service.sync"}
{}
wireless: starting communications {wait-module} {connecting}
wireless: modem now ON {modem-on}
wireless: obtaining essential configuration information from modem
wireless: waiting for wireless service {wait-service} {connecting}
wireless: waiting for wireless service 1 sec [----] {cell-registration-wait}
wireless: waiting for wireless service 3 sec [----] {cell-registration-wait}
wireless: waiting for wireless service 5 sec [----] {cell-registration-wait}
wireless: waiting for wireless service 8 sec [----] {cell-registration-wait}
wireless: waiting for wireless service 10 sec [++++] {cell-registration-wait}
wireless: waiting for wireless service 12 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 15 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 17 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 19 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 22 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 24 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 26 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 29 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 33 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 38 sec [+---] {cell-registration-wait}
wireless: waiting for wireless service 42 sec [+---] {cell-registration-wait}
wireless: cellular radio CAT-M1 band LTE BAND 28
wireless: waiting for wireless service 44 sec [+---] {cell-registration-wait}
wireless: waiting for data service {wait-data} {connecting}
wireless: network registration took 71 sec
wireless: connected {connected-closed}
project: using product UID product:com.XXX.demo
notehub: opened notehub {notehub-connected}
work: begin (anything pending) {sync-begin}
work: upload mydata.qo (1 changes) {sync-get-local-changes}
work: completed {sync-end}
notehub: closed notehub {notehub-disconnected}
wireless: modem now OFF (was active 73 sec, 1454 bytes transferred) {modem-off}
To my utter amazement, when I sent the sync command (which turns on the modem and tries to send your data to the Notehub cloud), it connected and within seconds I saw my data appear on Notehub! It’s nice to know that even with a small antenna (about the size of a Raspberry Pi A+ board), it performed as well as (if not better) than a mobile phone.
Logging into Notehub, I can see the full JSON blob from this message post:
{
"body": {
"sensor1": 6,
"units": "PM2.5 ug/m3"
},
"req": "note.add",
"when": 1588404575,
"routed": 1588404660,
"file": "mydata.qo",
"tower_country": "AU",
"tower_location": "McLaren Vale",
"tower_timezone": "Australia/Adelaide",
"tower_lat": -35.23553750000001,
"tower_lon": 138.523640625,
"tower_id": "XXX",
"device": "dev:XXX",
"product": "product:com.XXX.demo",
"session": "XXX",
"event": "XXX"
}
Looking at that data, you get the timestamp that your data point was created, the time it was routed to Notehub, as well as the location of the cell tower it used. Neat.
Sending data via Python
The next great thing about the Blues Notecard is that they have Python Bindings. This means that it should be quite easy to incorporate into my Enviro+ exporter code.
Because there isn’t a pip installable module for the Notecard yet, I decided to use git submodule to link their code to my codebase. This means that it’s really easy for me to pull in any future changes Blues make to the Notecard code into my codebase without having to copy/paste anything.
git submodule add https://github.com/blues/note-python.git notecard
This leads to the slightly awkward looking import at the top of my exporter, but much easier than updating manually. Here’s the code lifted out from my exporter that I’m using:
# Install the dependency:
# pip3 install python-periphery
import logging
import time
import notecard.notecard.notecard as notecard
from periphery import Serial
# Set the time between posts to 60 minutes (3600 seconds)
NOTECARD_TIME_BETWEEN_POSTS = '3600'
while True:
# The serial port that shows up when you connect
# the Notecard to the Raspberry Pi for me was /dev/ttyACM0
notecard_port = Serial("/dev/ttyACM0", 9600)
try:
card = notecard.OpenSerial(notecard_port)
except Exception as exception:
raise Exception("Error opening notecard: {}".format(exception))
# Send a data point to the Notecard queue
request = {'req':'note.add','body':{'PM1':16, 'units':'ug/m3'}}
try:
response = card.Transaction(request)
logging.info('Notecard response: {}'.format(response))
except Exception as exception:
logging.warning('Notecard data setup error: {}'.format(exception))
# Sync your Notecard queue with Notehub.io
request = {'req':'service.sync'}
try:
response = card.Transaction(request)
logging.info('Notecard response: {}'.format(response))
except Exception as exception:
logging.warning('Notecard sync error: {}'.format(exception))
# Wait 60 minutes until posting again
time.sleep(NOTECARD_TIME_BETWEEN_POSTS)
While testing, I reduced NOTECARD_TIME_BETWEEN_POSTS
down to 60 seconds so that I could see the data appear on Notehub.io quickly. But if you do that, don’t forget to switch it back to 3600 seconds (1 hour), or else you’ll use up all of your data credit in no time.
The only things I got stuck on while setting up were trying to find out which serial port the Notecard appeared at when connected to my Raspberry Pi. To do this I ran ls /dev/
before plugging it in, and then again after plugging it in and watched which one turned up. For me it was /dev/ttyACM0
.
I ran my code, and after a few little formatting bugs were fixed it was adding notes happily to the Notecard, and then syncing with Notehub.io a few seconds later.
Success!
So now I can run the entire setup off of my 15,000 mAh battery and get about 115 hours of uptime. That’s only ~4.5 days, so the next step is to look into something like a Sleepy Pi 2 to put it to sleep between readings, or add a Pijuice Solar charger.